In this post, I demonstate a debugging example from a university exercise I am working on. For context, this is about programming a linked list using class structures. The linked list is initialized with a pointer to it. The linked list class has member functions to append to, and print the linked list nodes.
A linked list consists of nodes; each node contains two units: data and a pointer to the 'next' node.
To be honest, the problem and solution actually turned out to be glaringly obvious, as you will spot soon! However, sometimes it is easy to overlook certain things that you would spot near-instantaneously 99% of the time. Hence, the purpose of this post lies with a sequence of debugging methods, rather than what the actual solution was. What's important is how the problem was found and addressed through the use of Visual Studio's debugging tools. Plus, the process only took a couple minutes.
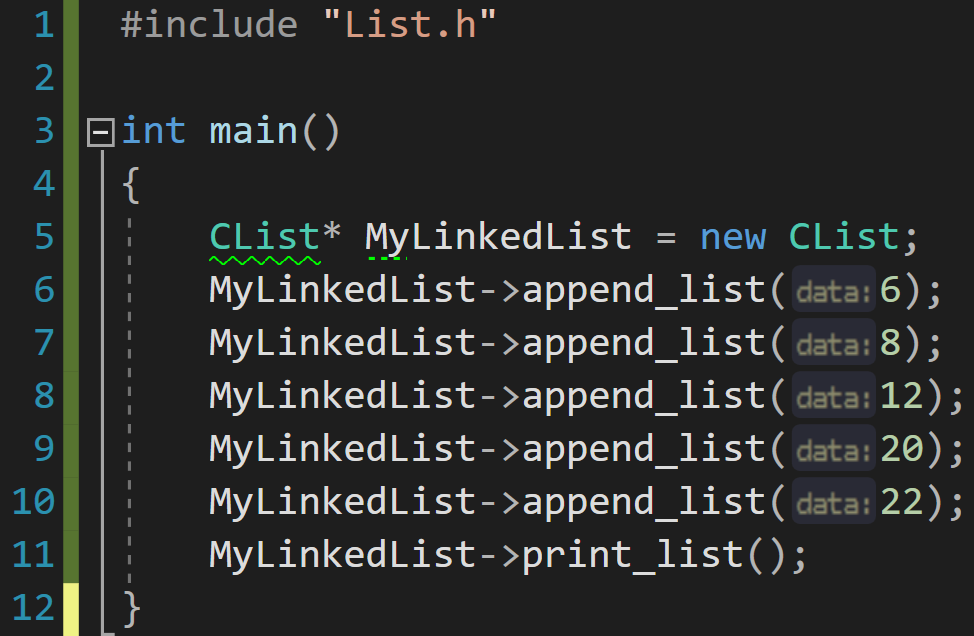
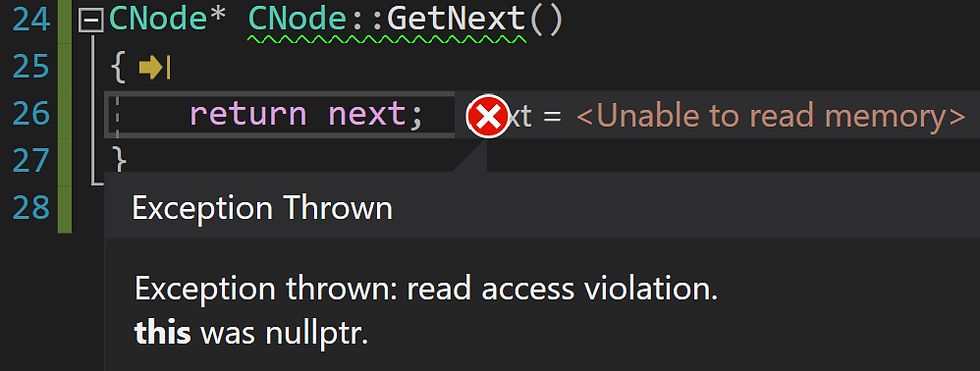
When ran in the debugger, this error is thrown.
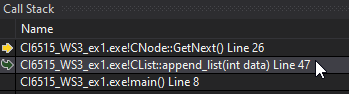
The call stack shows where the function, from which the error originated, was called.
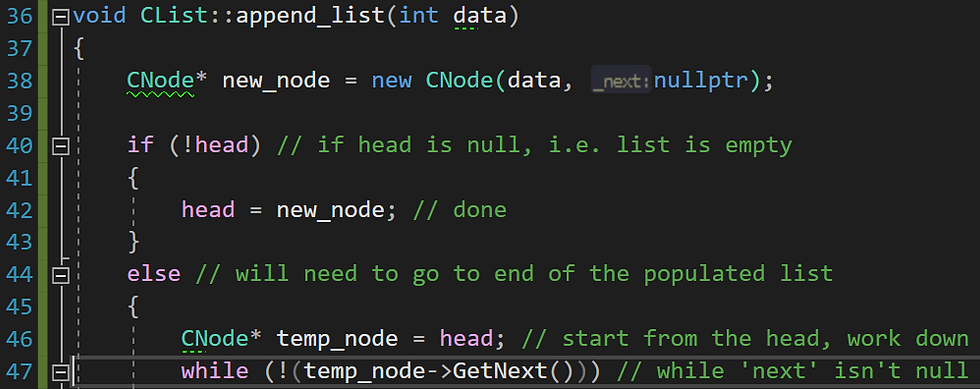
The error from 'GetNext' originates from the call of the 'append_list' function. As is visible from the main function, I try to call this multiple times to add values to the list. On reviewing the 'append_list' function, its purpose is to add data to the linked list. The if statement checks if the start node, known as the 'head' is null (empty). If the list is empty, the head is set to be a node containing the new data. The 'else' statement is only reached if the 'head' node exists and is populated with data.
Note that, on line 47, the conditional for the while statement does not correctly express the intentions specified by it's accompanying comment. This is where the obvious problem is that I happened to be blind to. Fortunately, this made for a better post on debugging!
For 'GetNext' to be called, this would require the list to already have a valid head node. To verify this, I can alter the main function:
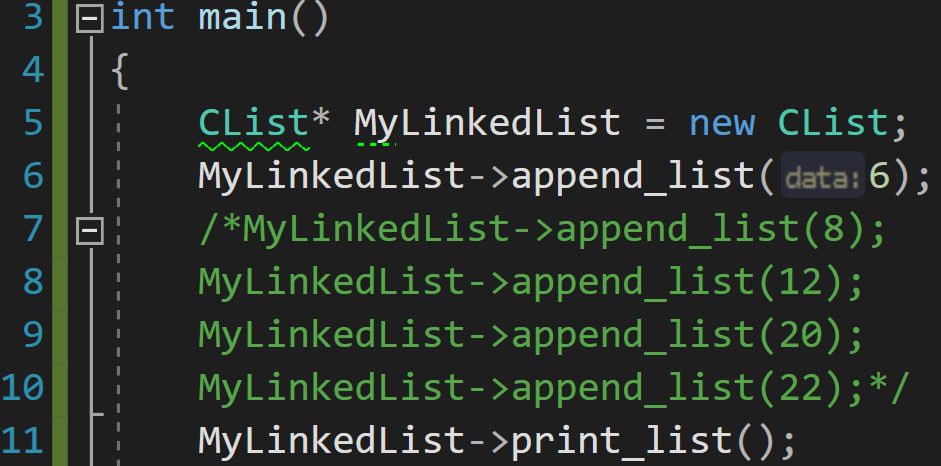
When ran, this only appends one entry to the linked list before printing the list out. The result is the number '6' being output to the console. This simply acts as proof of concept, to further validate the implication that the problem lies with the part of code shown in the call stack.
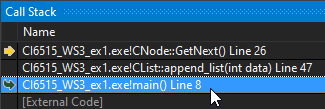
The call stack also directs you to the line of code which is meant to be executed when the previous line returns.
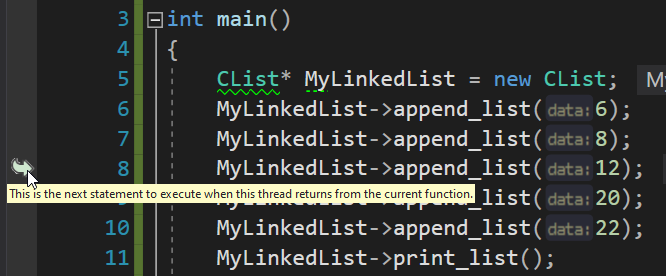
This is another quick way to see where things have gone wrong. This would imply that the problem is from line 7 (the function it calls).
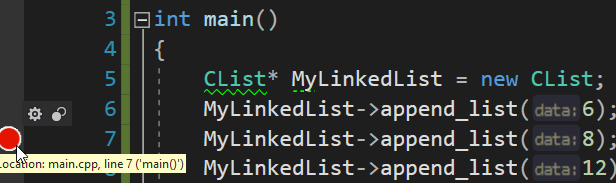
Line 6 works. After line 6 is complete, there will be a breakpoint before the second append is called.
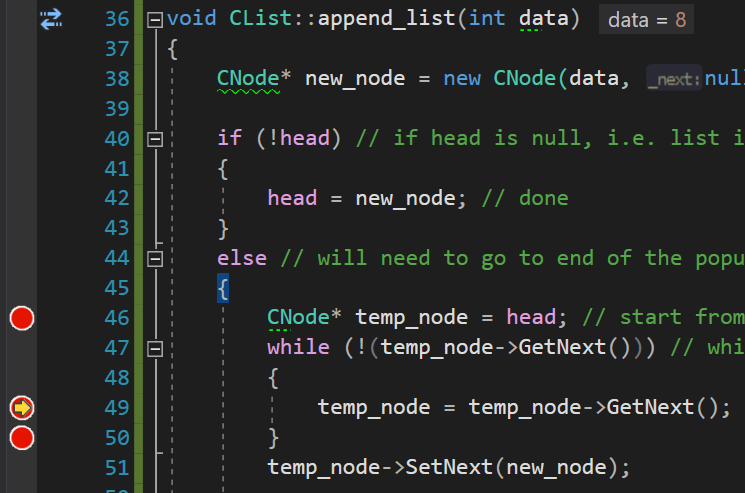
Breakpoints are set on the area of code I've narrowed the problem down to. After continuing across breakpoints, We reach line 49.

So far, checking the autos tab, we can verify that 'temp_node' has been set to be equivalent to 'head'. This is because the code intends to go through the list from start to finish, and begins at the head. Note that, so far, the value for 'next' is NULL. The intention of the condition is to check if 'next' is not 'NULL', meaning the loop hasn't reached the end of the list yet. As you can see, it does the opposite because of a logically incorrect conditional statement. But let's carry on, imagining that the statement isn't even visible, only using the debugging interface. How would we find the problem?

The operation on line 49 successfully executed, changing the value of 'temp_node'. Continuing past line 50's breakpoint shows the error shown in the beginning of the post.
Under the while loop's condition, considering that 'next' was null when it was being checked, it would imply that the conditional statement is incorrectly checking to see if NULL is returned. The line with the problem has now been pinpointed.
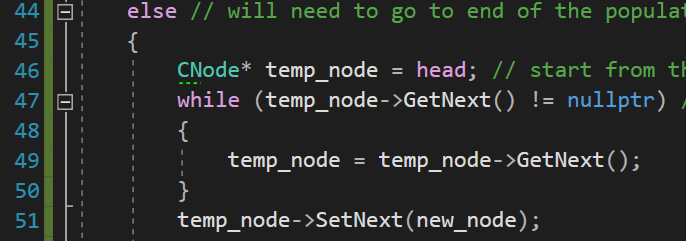
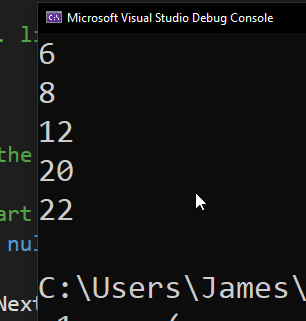
Success; the expected outcome has been met. The error was simply an error in the logic of the conditional statement.

The statement can also be written like so, simply removing the exclamation point.
Comments