Dynamic Delegates and Damage Fundamentals
- James Burke
- Sep 18, 2020
- 2 min read
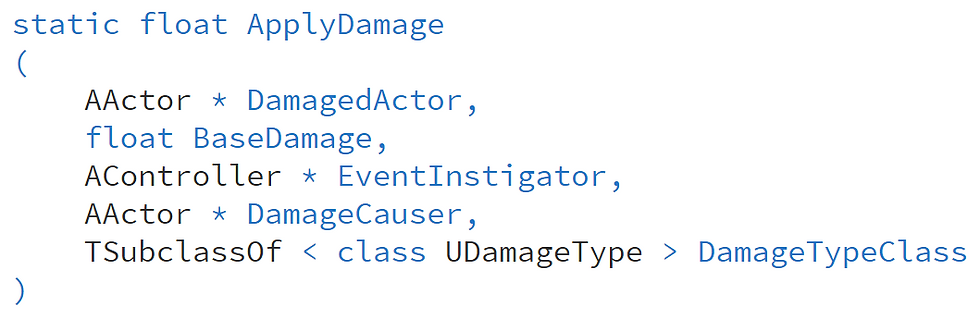
A dynamic delegate is an event which can be called or responded to. Whenever this event occurs, any listeners/recievers for the event will be able to respond by calling their own function. In this example, we have a base class for a projectile. We want to make it so that when there is collision, a function is called to register damage application and destroy the projectile actor.
In the header file (under the private access modifier), ensure the declarations under the 'Damage' category. For the damage type, in the editor, set this to be 'DamageType'.

We also need to declare the function. As this is a dynamic delegate function, this must be declared as a 'UFUNCTION()'. The reasoning behind all these parameters is that they are all required for a hit event. You can quickly view all the required parameters on a blueprint node for the equivalent event. Or, in VS: right click on 'OnComponentHit' (I'll show you where to type it soon, so when you do this you can right click it) > select 'Go to definition' > this opens PrimitiveComponent.h; CTRL + F 'OnComponentHit' > press enter. The five parameters after the name are what is required. It'll look like this:

Declare it; don't forget to generate the implementation,

Also, it is expected that this projectile already has a static mesh defined. The mesh contains the render and collision.
We can now go ahead with binding the event 'OnComponentHit' with the mesh of the projectile class which will be set to call 'OnHit' when the event occurs. In the constructor:

Now, in the actual function we will handle damage. Line 42 (and 43) specifically show this. Though, also ensure that you check for validity. This checks if the instigator of the projectile is valid, then checks for validity of the other actor (one being targeted), protects against any possible nested components of the same class, and also prevents the projectile damaging its source pawn (i,e, if the tank shoots, it will not damage itself directly by the projectile). Also, you need to make an include to use 'ApplyDamage'.

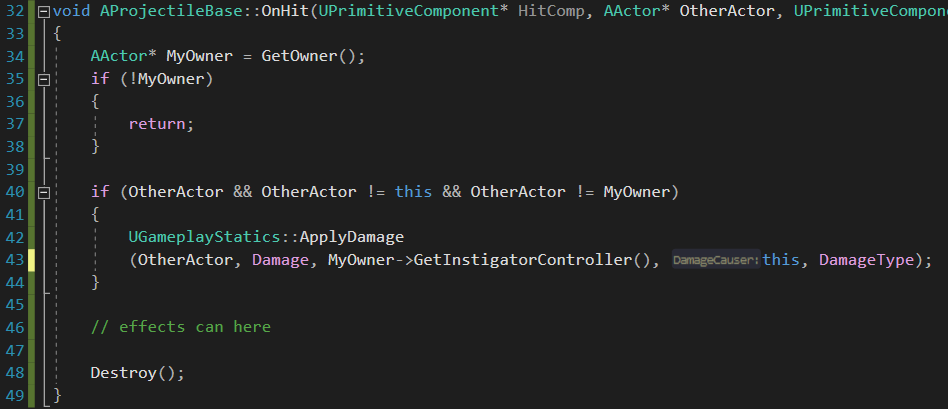
Also important to consider, the projectile must have a specified owner for these checks to work. This tends to be defined at the time it was spawned.
Comments