Performing Events Based on Elapsed Time
- James Burke
- Sep 15, 2020
- 2 min read
Updated: Sep 18, 2020
What for?
For when you want X to happen every Y seconds
Why this approach?
Rather than doing calculations constantly throughout each update with the tick function, which is unfavourable towards performance, using timers are beneficial for gameplay where you'd want something to happen over an elapsed time.
Example
In a game, I have a turret. I want this turret to fire a projectile every two seconds.
In the header file of the turret, I create a function which I want to be called every two seconds which will perform the desired behaviour. This will be a private function.
void CheckFireCondition();
In the CPP file, this is what the definition of my function is, shown below. The function, for demonstation, logs to the console. Now let's set this up to be called every two seconds.
void APawnTurret::CheckFireCondition()
{
UE_LOG(LogTemp, Warning, TEXT("Boom"));
}
We need to set a timer using the timer manager. This is the syntax. We need to initialise an FTimerHandle, we already have a function for the second parameter, we can define a float for the third.
void SetTimer
(
FTimerHandle & InOutHandle,
TFunction < void )> && Callback,
float InRate,
bool InbLoop,
float InFirstDelay
)
In the header file, define an FTimerHandle.
FTimerHandle FireRateTimerHandle;
Also define a float for how long the timer is. Make sure to set the appropiate access modifiers.
UPROPERTY(EditAnywhere, BlueprintReadWrite, Category ="Stats", meta = (AllowPrivateAccess = "true"))
float FireRate = 2.f;
I'd want the timer to begin when the turret is constructed. Therefore I will set up the timer on BeginPlay.
void APawnTurret::BeginPlay()
{
Super::BeginPlay();
GetWorldTimerManager().SetTimer(FireRateTimerHandle, this, &APawnTurret::CheckFireCondition, FireRate, true);
}
Then I have set the fifth parameter to true. As the syntax of the function shows, the value being 'true' sets the timer to loop.
The Result
The function, specified in the third parameter of the timer, is successfully called every two seconds, as proven by the console logs.
Another Quick Example
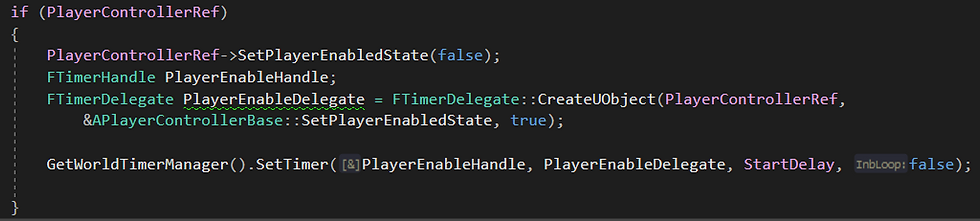
In this example, the situation differs in that we create a delegate to use in the parameter. This is because I need a function from a different class. In this function I'd want to pass it with a value of 'true'. The function call indicated by the delegate occurs once the timer reaches the value provided by 'StartDelay'.
Comentarios