Inheritance, Polymorphism, and more
- James Burke
- Sep 25, 2020
- 3 min read
Updated: Sep 26, 2020
Github
Description
This post is an overview of a workshop task from uni. In this post I show C++ programming for:
Inheritance
Polymorphism
Memory-leak prevention
Accessing real-world local time
This exercise was to practice object oriented principles in the example context of bank accounts. The aim was not to build a bank system, but rather use the context of bank accounts to express OOP knowledge. The same could be done for other contexts, such as shapes or animals, to name a few.
File structure
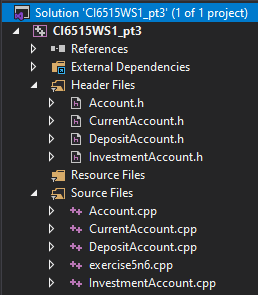
I personally decided to organise the project by having header files for each class, with cpp files for implementation of functions and whatnot. From prior experience with Unreal Engine, this way of organisation came as natural.
Class diagram
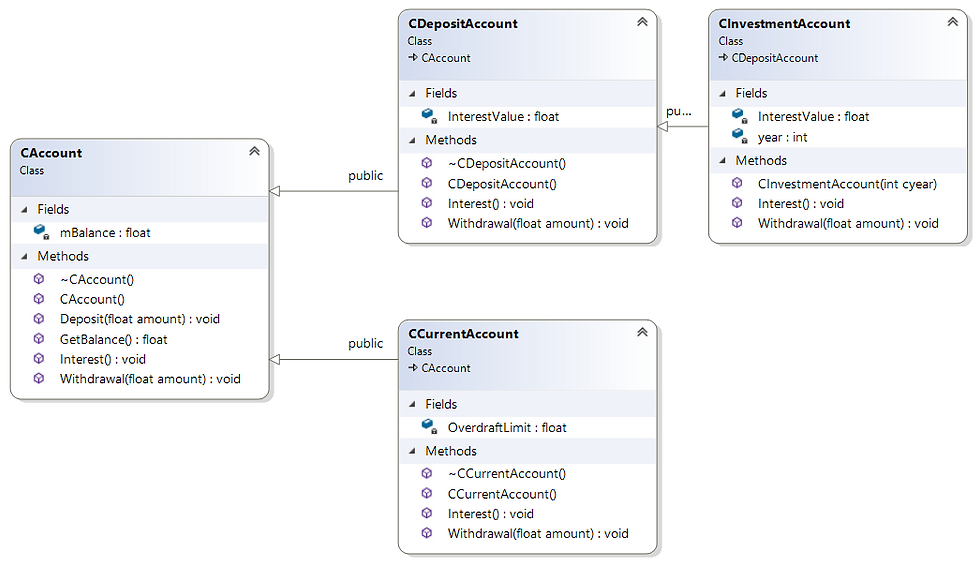
As not explicitly shown in the diagram, the functions 'Interest' and 'Withdrawal' are virtual functions. They are declared as such to let the editor know that derived functions intend to override them with specific implementations.
Code walkthrough
In addition to the classes shown above, there is a cpp file responsible for initialising these classes and performing tests for console logs, this is called 'exercise5n6.cpp'.
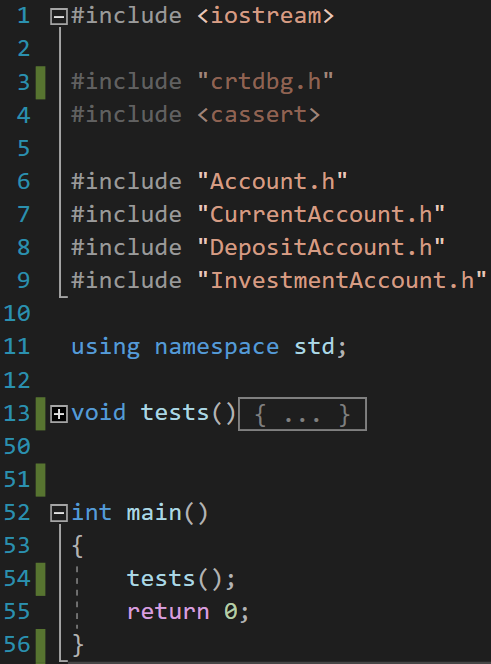
line 1: include for I/O capabilities as it is required to do console logs
line 3: will be for detecting memory leaks
line 4: ignore this, it's removed
lines 6 - 9: includes for each header file of the classes
line 13: function where all the tests of functionalities are encapsulated
line 52: main function which runs at the beginning of runtime
Now, let's go through the 'tests' function.
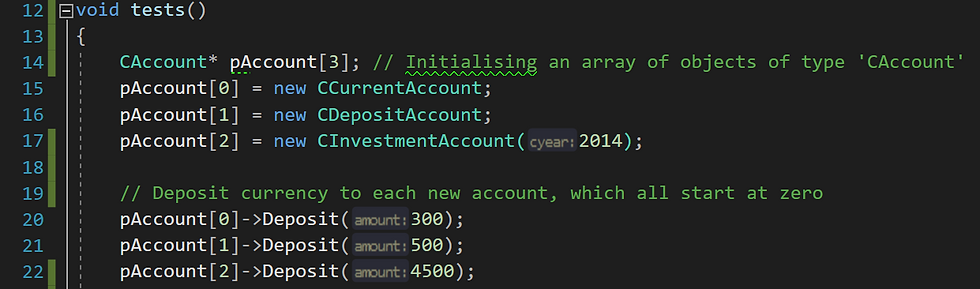
I begin with initialising a pointer array of objects of type 'CAccount', which is the base class of each class in this code. Initialising these derived classes under the base class unifies them. I then have three lines for depositing each type. Now this wouldn't be efficient if I had, say, one hundred instances, but in this code there are only three for the purpose of testing.
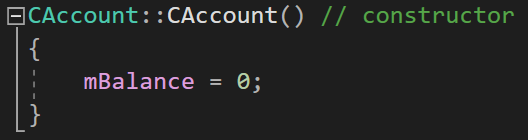
Looking at the constructor of the base class, it shows that initial balance will be zero.
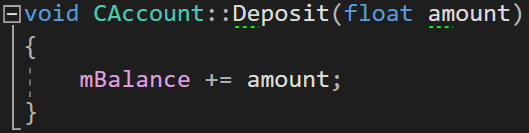
Deposit function is defined and implemented in the base class. It is public, so the derived classes will call this function from the base class. This function directly changes the balance. You'll notice the withdraw function is defined as virtual in the base class, and only has implementations in the base classes, where this deposit function is called from the withdraw functions, supplied with a negative value to achieve a deduction in balance.
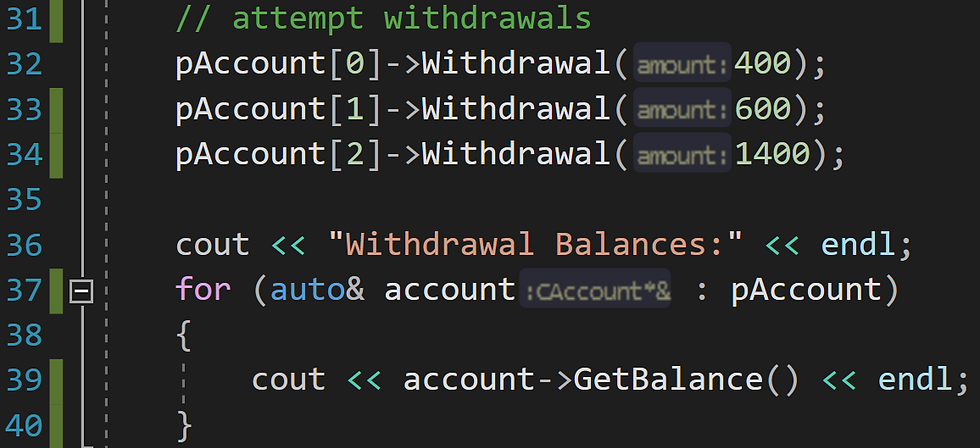
To summarise each 'Withdrawal' implementation:
CCurrentAccount: withdrawal occurs if it meets the condition that the balance after the withdraw will not be negative, i.e. go below zero. This also considers that an overdraft limit is applied.
CDepositAccount: same as above, but without consideration of an overdraft limit
CInvestmentAccount: gets the current time, specifically the year, and checks to see if the year minus the specified year is equal to or greater than 2. This is because withdrawals on the investment account cannot happen unless it has been 2 years since the previous withdrawal. This is why CInvestmentAmount takes an argument at initialisation.
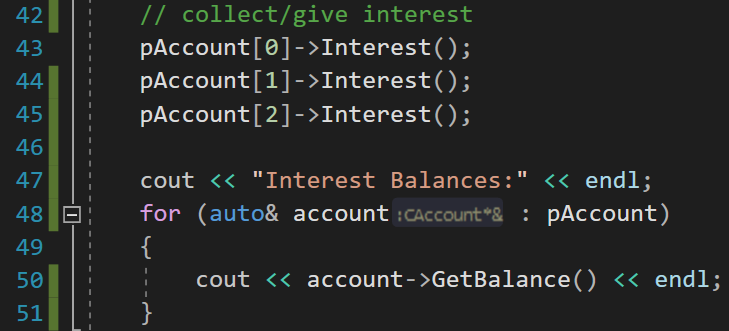
To summarise interest implementations, both CInvestmentAccount and CDepositAccount add interest if the balance is greater than zero. CCurrentAccount only takes interest from the balance when it is below zero (debt interest).
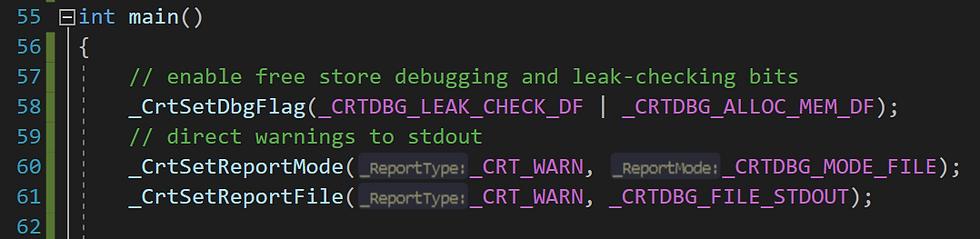
This wasn't in the previous screenshot but I just added it. This make it so that memory-leak detection is enabled, and if it is detected then warnings will show in the console. I will also paste it here for future reference:
#include "crtdbg.h"
// enable free store debugging and leak-checking bits
_CrtSetDbgFlag(_CRTDBG_LEAK_CHECK_DF | _CRTDBG_ALLOC_MEM_DF);
// direct warnings to stdout
_CrtSetReportMode(_CRT_WARN, _CRTDBG_MODE_FILE);
_CrtSetReportFile(_CRT_WARN, _CRTDBG_FILE_STDOUT);
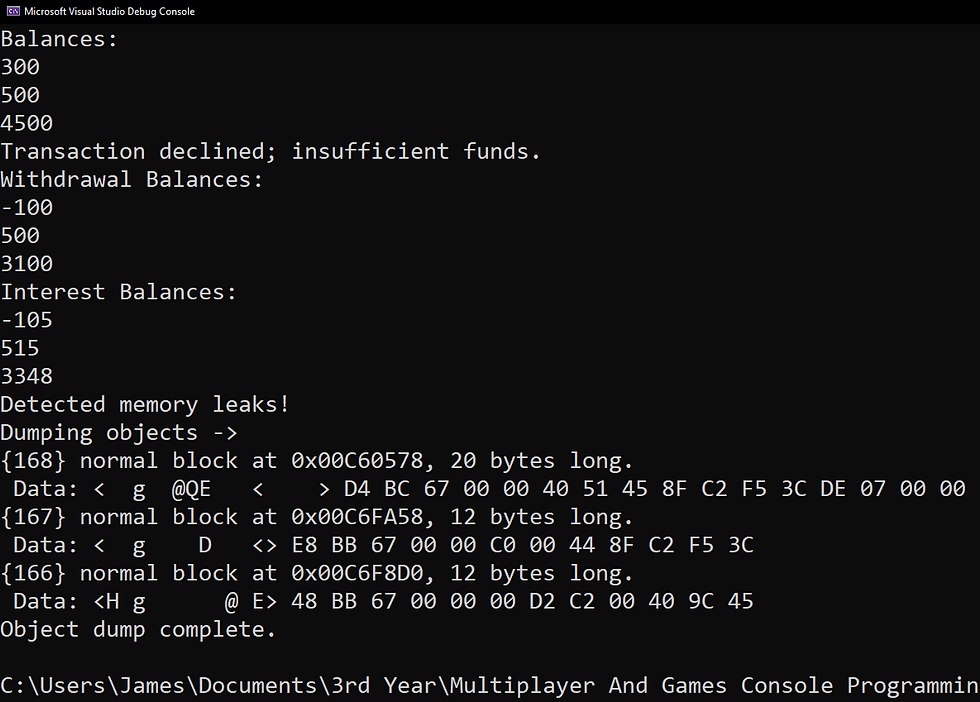
On testing each function, I found no semantic errors. Everything works as intended. Note that memory leaks have been detected. This is simply alleviated by adding this to the end of our 'tests' function.

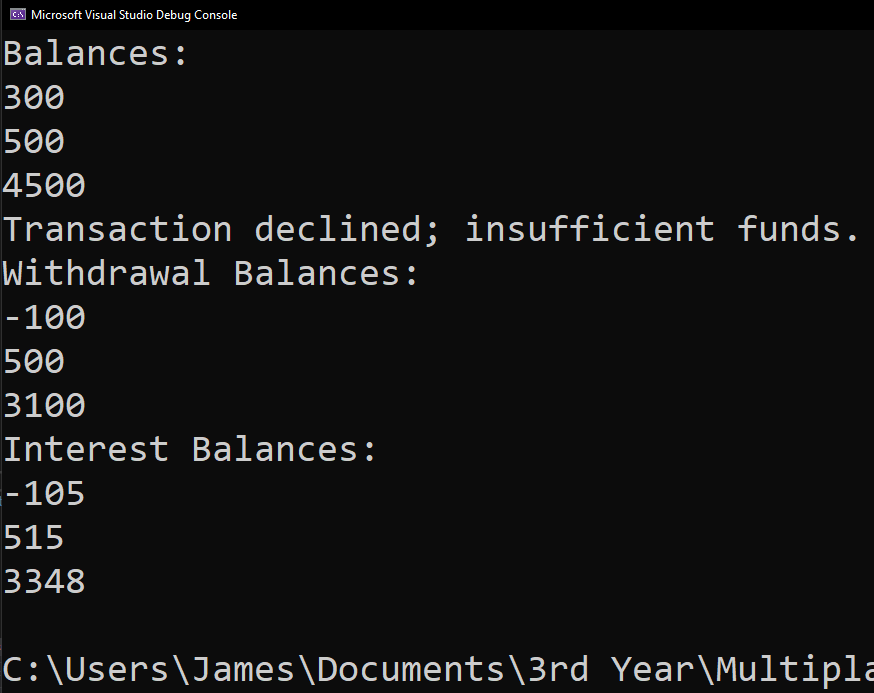
Now there are no warnings when ran. Nice. To explain, memory leaks occur when the program fails to release allocated memory. In this case, the memory for the pointers of the objects. 'delete' simple releases the memory at a specified address. It typically calls the 'free' function, but this happens under-the-hood. It also can invoke the destructor of a class, if it exists.
As an extra, this is an example on how to ge the time using 'localtime_s' rather than 'localtime'. I use the '_s' variant as microsoft declared the latter as deprecated, so I hads to find out how to use the former.
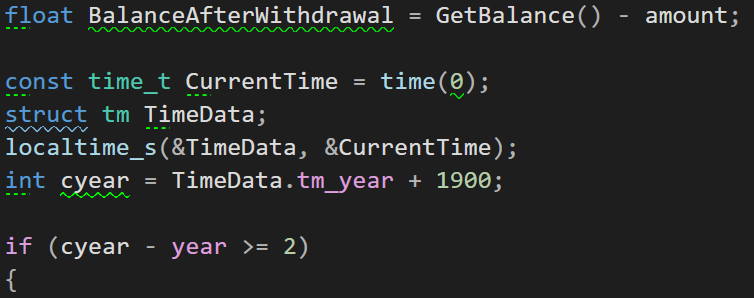
Comments