A video to show the results of implementation:
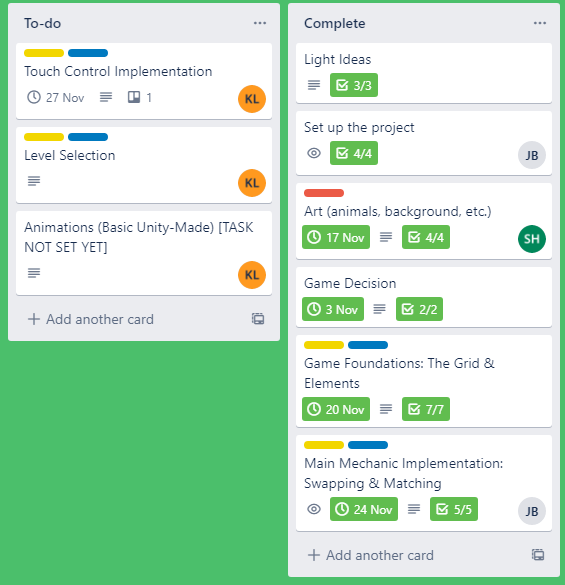
From our Trello board, this is our current progress and what's next. In the previous post I completed the following regarding the grid and it's elements:
Grid alignment and scaling
Randomization of grid elements
Falling of grid elements to fill space
An obstacle as an extra element type
Now that these have been finished, I moved on to developing the main mechanics for swapping elements to create matches.
This week, I implemented:
Swapping, based on valid 'matching' conditions
Removal of elements when matched
Filling the board after a match is made
Criteria for making matches:
Minimum of 3 elements of the same appearance
Horizontal or vertical
L and T shaped matches should work
How elements are cleared
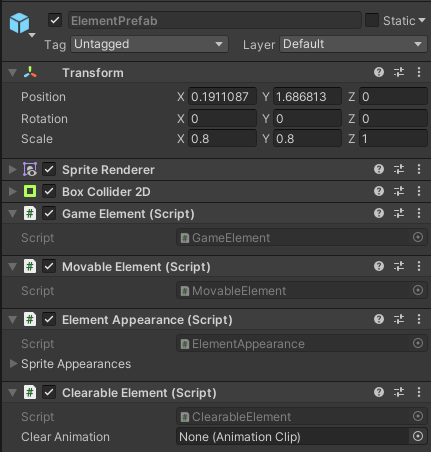
I decided to create a script to attach to anything which can be cleared off the board- this way I can easily reuse code to apply behaviours to multiple game objects, if I need to in the future. See "Clearable Element"- an animation can be set which will be played before an element is cleared, too. This will be included in the future- for now, there is no clearance animation, but the element is simply destroyed after it is moved to it's new position.
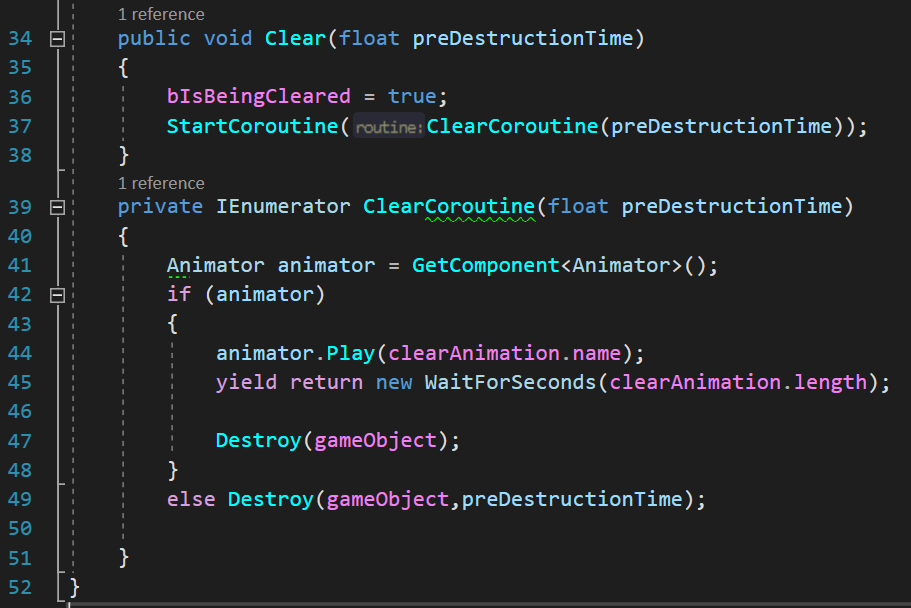
Clearing an element is called as a coroutine where, if there is an animation supplied, the duration of the animation is waited for before the element is destroyed.
Input Functions
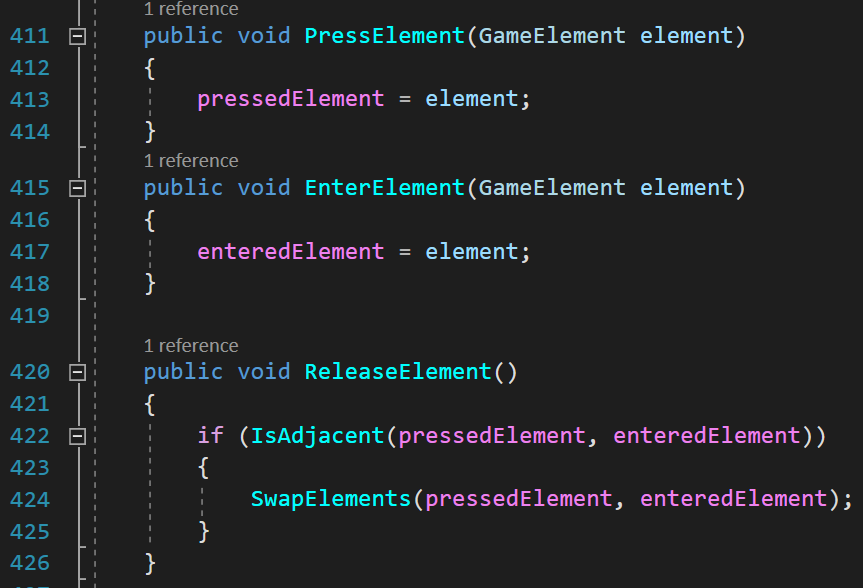
The GameElement script handles direct input. Containing a reference to the board manager script, the mouse input functions call these functions in BoardManager. To summarise: player taps element > player drags to select an adjacent element > player releases press > game initiates a swap of the two elements.
Swapping Elements
The "SwapElements" function houses its own logic and calls to four other functions I have implemented: "GetMatch" houses the logic for detecting matches between elements; "Move" (of the "MovableComponent" script) facilitates the visual aspect of element swapping; "ClearValidMatches" ensures that all elements which are matched - even inadvertantly - are cleared; "Fill" is ran as a coroutine- it ensures that all empty spaces are filled after matches are made.
A summary of how matches are determined (the code is over 100 lines long at the moment):
ITERATE horizontally:
COMPARE Appearances (from appearance component) of the adjacent
cells
IF they MATCH in appearance, ADD to list X(horizontal matches)
END OF ITERATIONS
IF matches were found:
RETURN list X
IF the LENGTH of the list X is GREATER THAN 2:
ADD the list to list Z(contains matching elements)
FOR EACH element of list Z:
TRAVERSE vertically to find potential matches (L / T shaped)
Do the same as previous in adding to list Z
IF no horizontal matches were found:
ITERATE vertically:
Repeat the same operations above, except from vertical direction,
followed by further traversal for L and T shaped matches.
END OF ITERATIONS
IF matches were found:
RETURN list X
Comments